The following are php snippets to add to any website.
Gutenberg
//Adds Reusable Block FW and Elements Fixes
add_action( 'enqueue_block_editor_assets', function() {
$css = '
.wp-block.is-reusable{max-width: 100%;}
.post-type-gp_elements .is-root-container>.wp-block[data-align=wide]{max-width:1500px;}
.post-type-gp_elements .is-root-container>.wp-block[data-align=full]{max-width:100%;}
.post-type-gp_elements .is-root-container>.wp-block{max-width: calc(1500px - 20px - 20px);}
';
wp_add_inline_style( 'generate-block-editor-styles', $css );
}, 100 );
//Disable Gutenberg for Post Types
function my_disable_gutenberg( $current_status, $post_type ) {
// Disabled post types
$disabled_post_types = array( 'review');
// Change $can_edit to false for any post types in the disabled post types array
if ( in_array( $post_type, $disabled_post_types, true ) ) {
$current_status = false;
}
return $current_status;
}
add_filter( 'use_block_editor_for_post_type', 'my_disable_gutenberg', 10, 2 );
GeneratePress
//Enable Columns on Custom Post Types
add_filter( 'generate_blog_columns','tu_portfolio_columns' );
function tu_portfolio_columns( $columns ) {
if ( is_tax( 'productcategory' ) ) {
return true;
}
return $columns;
}
add_filter( 'generate_blog_columns','tu_portfolio_columns2' );
function tu_portfolio_columns2( $columns ) {
if ( is_archive( 'product' ) ) {
return true;
}
return $columns;
}
Example Custom Post Type
Can also use this link
// Product Post
function custompost_product() {
$labels = array(
'name' => _x( 'PPE Gear Dryers', 'Post Type General Name', 'text_domain' ),
'singular_name' => _x( 'Products', 'Post Type Singular Name', 'text_domain' ),
'menu_name' => __( 'Products', 'text_domain' ),
'name_admin_bar' => __( 'Product', 'text_domain' ),
'archives' => __( 'Product Archives', 'text_domain' ),
'attributes' => __( 'Product Attributes', 'text_domain' ),
'parent_item_colon' => __( 'Parent Product:', 'text_domain' ),
'all_items' => __( 'All Products', 'text_domain' ),
'add_new_item' => __( 'Add New Product', 'text_domain' ),
'add_new' => __( 'Add New', 'text_domain' ),
'new_item' => __( 'New Product', 'text_domain' ),
'edit_item' => __( 'Edit Product', 'text_domain' ),
'update_item' => __( 'Update Product', 'text_domain' ),
'view_item' => __( 'View Product', 'text_domain' ),
'view_items' => __( 'View Products', 'text_domain' ),
'search_items' => __( 'Search Product', 'text_domain' ),
'not_found' => __( 'Not found', 'text_domain' ),
'not_found_in_trash' => __( 'Not found in Trash', 'text_domain' ),
'featured_image' => __( 'Featured Image', 'text_domain' ),
'set_featured_image' => __( 'Set featured image', 'text_domain' ),
'remove_featured_image' => __( 'Remove featured image', 'text_domain' ),
'use_featured_image' => __( 'Use as featured image', 'text_domain' ),
'insert_into_item' => __( 'Insert into product', 'text_domain' ),
'uploaded_to_this_item' => __( 'Uploaded to this product', 'text_domain' ),
'items_list' => __( 'Products list', 'text_domain' ),
'items_list_navigation' => __( 'Products list navigation', 'text_domain' ),
'filter_items_list' => __( 'Filter Products list', 'text_domain' ),
);
$args = array(
'label' => __( 'PPE Dryers Products', 'text_domain' ),
'description' => __( 'PPE Dryers Products', 'text_domain' ),
'labels' => $labels,
'supports' => array( 'title', 'custom-fields','thumbnail' ),
'taxonomies' => array( '' ),
'hierarchical' => false,
'public' => true,
'show_ui' => true,
'show_in_menu' => true,
'menu_position' => 5,
'menu_icon' => 'dashicons-cart',
'show_in_admin_bar' => true,
'show_in_rest' => true,
'show_in_nav_menus' => true,
'can_export' => true,
'has_archive' => 'fire-gear-dryers',
'exclude_from_search' => false,
'rewrite' => array( "slug" => "dryers/%productcategory%", "with_front" => false ),
'publicly_queryable' => true,
'capability_type' => 'page',
);
register_post_type( 'product', $args );
}
add_action( 'init', 'custompost_product', 0 );
Comments
//Remove Comments
add_action('admin_init', function () {
// Redirect any user trying to access comments page
global $pagenow;
if ($pagenow === 'edit-comments.php' || $pagenow === 'options-discussion.php') {
wp_redirect(admin_url());
exit;
}
// Remove comments metabox from dashboard
remove_meta_box('dashboard_recent_comments', 'dashboard', 'normal');
// Disable support for comments and trackbacks in post types
foreach (get_post_types() as $post_type) {
if (post_type_supports($post_type, 'comments')) {
remove_post_type_support($post_type, 'comments');
remove_post_type_support($post_type, 'trackbacks');
}
}
});
// Close comments on the front-end
add_filter('comments_open', '__return_false', 20, 2);
add_filter('pings_open', '__return_false', 20, 2);
// Hide existing comments
add_filter('comments_array', '__return_empty_array', 10, 2);
// Remove comments page and option page in menu
add_action('admin_menu', function () {
remove_menu_page('edit-comments.php');
remove_submenu_page('options-general.php', 'options-discussion.php');
});
// Remove comments links from admin bar
add_action('add_admin_bar_menus', function () {
if (is_admin_bar_showing()) {
remove_action('admin_bar_menu', 'wp_admin_bar_comments_menu', 60);
}
});
Custom Taxonomy/Category
//Product Category
add_action( 'init', 'build_taxonomy1', 0 );
function build_taxonomy1() {
register_taxonomy(
'productcategory',
'product',
array(
'hierarchical' => true,
'label' => 'Product Category',
'query_var' => true,
'rewrite' => array( "slug" => "dryers", "with_front" => false ),
)
);
}
//Add Category Slug to Single Post
add_filter('post_type_link', 'cj_update_permalink_structure', 10, 2);
function cj_update_permalink_structure( $post_link, $post )
{
if ( false !== strpos( $post_link, '%productcategory%' ) ) {
$taxonomy_terms = get_the_terms( $post->ID, 'productcategory' );
foreach ( $taxonomy_terms as $term ) {
if ( ! $term->parent ) {
$post_link = str_replace( '%productcategory%', $term->slug, $post_link );
}
}
}
return $post_link;
}
//Shortcode for a Post List Menu with Active Category Open
function categorylist_shortcode(){
$categories = get_terms( array(
'taxonomy' => 'productcategory',
'hide_empty' => false,
'orderby' => 'name',
'order' => 'DES'
) );
$terms = get_the_terms( $post->ID , 'productcategory' );
$term = array_shift( $terms );
$termid = $term->term_id;
$returnhtml = '<div class="productmenu">';
foreach( $categories as $category ) {
if($category->term_id == $termid){
$returnhtml .= '<h3 class="currentcategory"><a href="' . get_term_link($category->term_id) . '">' . $category->name . '</a></h3>';
$args = array(
'post_type' => 'product',
'tax_query' => array(
array('taxonomy' => 'productcategory','field' => 'id','terms' => $termid,),),
);
$loop = new WP_Query( $args );
if ( $loop ->have_posts() ) {
$returnhtml .= "<ul>";
while ( $loop ->have_posts() ) {
$loop ->the_post();
$returnhtml .= '<li><a href="'.get_permalink().'">'.get_the_title().'</a></li>';
}
$returnhtml .= "</ul>";
wp_reset_postdata(); // reset to avoid conflicts
} else {
}
}
else{
$returnhtml .= '<h3><a href="' . get_term_link($category->term_id) . '">' . $category->name . '</a></h3>';
}
}
$returnhtml .= '</div>';
return $returnhtml;
}
add_shortcode('categorylist','categorylist_shortcode');
SEO Framework CPT Home/Main Archive
//Title
add_filter( 'the_seo_framework_title_from_generation', function( $title, $args ) {
if ( null === $args && is_post_type_archive( 'product' ) ) {
$title = 'Turnout Gear Dryers for Fire Departments | Williams Direct Dryers';
}
return $title;
}, 10, 2 );
//Remove Site Title
add_filter( 'the_seo_framework_use_title_branding', function( $use ) {
if ( null === $args && is_post_type_archive( 'product' ) ) {
$use = false;
}
return $use;
} );
//Description
add_filter( 'the_seo_framework_generated_description', function( $description, $args ) {
if ( null === $args && is_post_type_archive( 'product' ) ) {
$description = 'Williams Direct Dryers prides itself on quality. View our selection of dryers engineered for turnout gear, EOD suits, hazmat suits, SCBA masks, and more.';
}
return $description;
}, 10, 2 );
Custom Login Page/Enqueue Style/Script
function av_login_stylesheet() {
wp_enqueue_style( 'custom-login', '/wp-content/styles/style-login.css' );
}
add_action( 'login_enqueue_scripts', 'av_login_stylesheet' );
function av_login_scripts() {
wp_enqueue_script( 'custom-login.js', '/wp-content/scripts/script-login.js', array( 'jquery' ), 1.0 );
}
add_action( 'login_enqueue_scripts', 'av_login_scripts', 10 );
Woocommerce
//Display Woocommerce Price Shortcode
add_shortcode( 'sc_product_price', 'woo_product_price_shortcode' );
function woo_product_price_shortcode( $atts ) {
$atts = shortcode_atts( array(
'id' => null,
'append' => null,
), $atts, 'sc_product_price' );
if ( empty( $atts[ 'id' ] ) ) {
return '';
}
$append = $atts['append'];
$product = wc_get_product( $atts['id'] );
if ( ! $product ) {
return '';
}
return $product->get_price_html() . $append;
}
//Change Add to Cart Text
add_filter( 'woocommerce_product_single_add_to_cart_text', 'av_custom_single_add_to_cart_text' );
function av_custom_single_add_to_cart_text(){
global $product;
if ($product->get_stock_status() !== 'instock'){
return __( 'Out of Stock', 'woocommerce' );
} else {
return __( 'Add to Cart', 'woocommerce' );
}
}
//Remove Additional Information Tab
add_filter( 'woocommerce_product_tabs', 'my_remove_product_tabs', 98 );
function my_remove_product_tabs( $tabs ) {
unset( $tabs['additional_information'] ); // To remove the additional information tab
return $tabs;
}
//Remove Product Title
function product_change_title_position() {
remove_action( 'woocommerce_single_product_summary', 'woocommerce_template_single_title', 5 );
}
add_action( 'init', 'product_change_title_position' );
//Remove Variation Clear Text
add_filter('woocommerce_reset_variations_link', '__return_empty_string');
//Custom Append to Variable Product Prices
function wc_varb_price_range( $wcv_price, $product ) {
$prefix = sprintf('%s: ', __('Starting at', 'wcvp_range'));
$wcv_reg_min_price = $product->get_variation_regular_price( 'min', true );
$wcv_min_sale_price = $product->get_variation_sale_price( 'min', true );
$wcv_max_price = $product->get_variation_price( 'max', true );
$wcv_min_price = $product->get_variation_price( 'min', true );
$wcv_price = ( $wcv_min_sale_price == $wcv_reg_min_price ) ?
wc_price( $wcv_reg_min_price ) :
'<del>' . wc_price( $wcv_reg_min_price ) . '</del>' . '<ins>' . wc_price( $wcv_min_sale_price ) . '</ins>';
return ( $wcv_min_price == $wcv_max_price ) ?
$wcv_price :
sprintf('%s%s', $prefix, $wcv_price);
}
add_filter( 'woocommerce_variable_sale_price_html', 'wc_varb_price_range', 10, 2 );
add_filter( 'woocommerce_variable_price_html', 'wc_varb_price_range', 10, 2 );
//Product Addons Fix for Many Variations
if (!function_exists('custom_wapo_conditional_logic_limit')) {
function custom_wapo_conditional_logic_limit($limit) {
return 200;
}
add_filter( 'yith_product_variations_chosen_list_limit', 'custom_wapo_conditional_logic_limit', 99, 1 );
}
Common Use Cases
Remove author links in blog posts. Eg. format: https://www.mid-americagolf.com/author/admin/
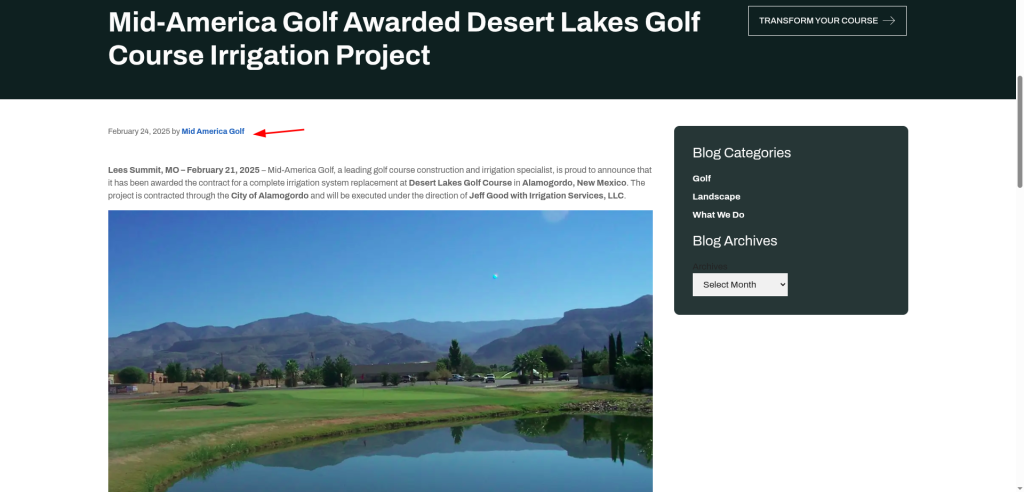
//disable author links in blog posts from showing & indexing in code
add_filter( 'generate_post_author_link', '__return_false' );
If this php snippet doesn’t work (sometimes incompatible with GeneratePress or latest Gutenberg or Bricks), then the template file needs to be edited.
For GeneratePress, you have to take this file (post-meta.php) and copy it over to the child theme:
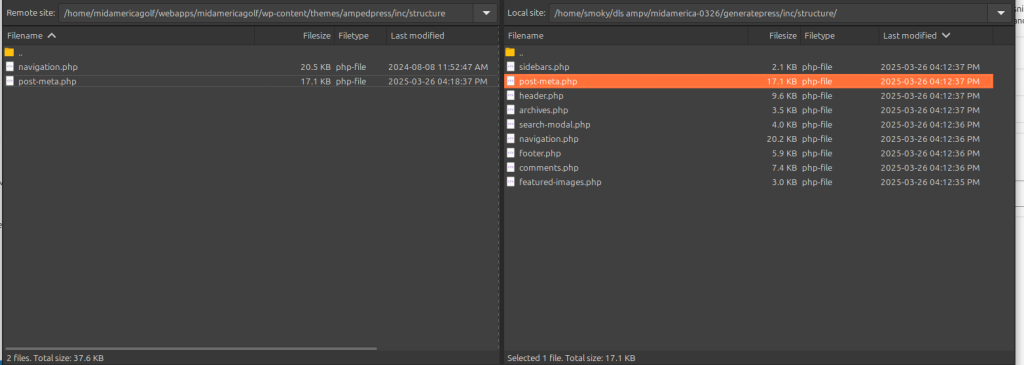
Then open it and edit this line of code and just set this to “false” to display the author name without a link:
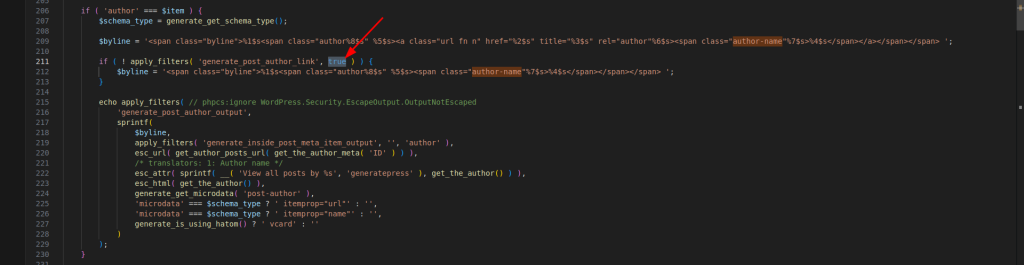
Other use cases, non-GeneratePress try this snippet:
function disable_author_posts_link( $output ) {
return strip_tags( $output );
}
add_filter( 'the_author_posts_link', 'disable_author_posts_link' );
Or
function disable_author_posts_link( $link ) {
return get_the_author();
}
add_filter( 'the_author_posts_link', 'disable_author_posts_link' );
Dynamic Expiration Date
If we need to add a coupon expiration date or any other dynamic date in our content like so:
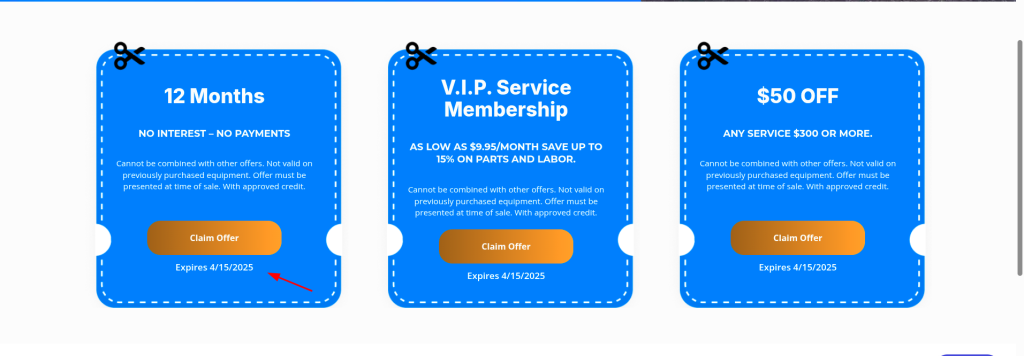
Add the following span:
<span class="dynamic-expiration-date"></span>
With this js added to /js/ as so:
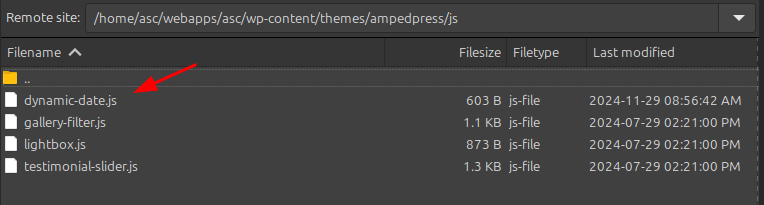
With this js code (just make sure the class matches the span class ‘.dynamic-expiration-date’) and configure the formula to make it any period (set to 2 weeks by default):
document.addEventListener('DOMContentLoaded', () => {
const expirationElements = document.querySelectorAll('.dynamic-expiration-date');
expirationElements.forEach(element => {
const today = new Date();
const nextMonth = today.getDate() > 15 ? today.getMonth() + 1 : today.getMonth();
const year = nextMonth === 0 ? today.getFullYear() + 1 : today.getFullYear();
const expirationDate = new Date(year, nextMonth, 15);
const formattedDate = expirationDate.toLocaleDateString('en-US');
element.textContent = `Expires ${formattedDate}`;
});
});